Game development is intrinsically entwined with mathematics, as a multitude of mathematical computations play a pivotal role throughout the game development process. Within the Unity game engine, an indispensable tool emerges known as Mathf, encompassing a comprehensive array of mathematical functions essential for crafting your game. Remarkably, Mathf seamlessly integrates within the Unity engine’s namespace, sparing you from any need for additional integration.
The Mathf library boasts an expansive array of static functions, with a comprehensive compendium readily accessible within Unity’s documentation. For the scope of this tutorial, our focus centers on the frequently utilized Mathf.Clamp function, illuminated through practical examples.
Within the Unity environment, the Mathf.Clamp function serves to confine the value of both Floats and Integers within specified boundaries. This versatile function takes three parameters into account. The first parameter designates the variable in question, the second parameter denotes the lower limit, and the third parameter signifies the upper boundary.
When the variable’s value falls short of the lower limit, Mathf.Clamp returns the minimum threshold value. Conversely, if the variable surpasses the upper limit, the function yields the maximum range value. Should the variable fall within the designated range, the output mirrors the variable’s original value.
Clamping Float Values in Unity Using C#
In game development, there are instances when there’s a need to restrict a variable’s value within a specific range. In Unity, this is achieved using the Mathf.Clamp function. Here’s a detailed and comprehensive guide to understand and implement this concept:
Copy code
using UnityEngine;
public class FloatClampExample : MonoBehaviour
{
// Initial float value
float currentValue = 0f;
// Defined range for clamping
float minValue = 10.0f;
float maxValue = 20.0f;
// The Update method is called once per frame in Unity
void Update()
{
// Increment the current value
currentValue += 1.0f;
// Clamp the currentValue to make sure it remains between minValue and maxValue
currentValue = Mathf.Clamp(currentValue, minValue, maxValue);
// Log the current value for debugging purposes
Debug.Log(currentValue);
}
}
In this script, we start with an initial currentValue of 0f. Every frame (during the game’s runtime), the Update method is called, increasing the currentValue by 1.0f. The Mathf.Clamp function then ensures that the currentValue never goes below minValue or above maxValue. Thus, even if the currentValue theoretically increases beyond 20 due to the increment operation, the clamped value will always be 20.
Understanding the Output:
When the script runs, the output logged to the console will start from 10 (as the initial value of 0 is clamped to the minimum value of 10). It will increment by 1 every frame until it reaches 20. From then onwards, even though the script continues to add 1.0f to the currentValue, the output will consistently be 20 because the value has been clamped.
Quick Tip for Clamping Between 0 and 1:
In scenarios where there’s a need to restrict a float value specifically between 0 and 1, Unity provides a shorthand method: Mathf.Clamp01. This method is especially useful for normalizing values.
Usage:
float result = Mathf.Clamp01(someFloatValue);
The Mathf.Clamp01 function is streamlined for this common case, taking a single input variable and automatically clamping its value between 0 and 1. It’s an efficient way to ensure values remain within a normalized range, especially useful when dealing with percentages, lerp operations, or any functionality requiring values between 0 and 1.
Clamping Vector3 Values in Unity: A Comprehensive Guide
In Unity, when dealing with the Vector3 data structure, there are multiple ways to restrict or “clamp” its values. Each axis of the Vector3 data structure – X, Y, and Z – represents a different dimension in the 3D space. Depending on the desired outcome, you might want to restrict the entire Vector3 magnitude or clamp each individual axis separately.
1. Clamping the Magnitude of Vector3
Unity offers the Vector3.ClampMagnitude method to limit the magnitude of a Vector3. This method adjusts the length of the Vector3 without changing its direction.
2. Clamping Individual Axes: A Practical Example
For a more granular approach, you can clamp each axis separately using the Mathf.Clamp method. Here’s a step-by-step guide to clamp a game object’s position along the Y-axis:
- Script Creation: Start by creating a new script named vector_clamp;
- Integration with GameObject: Attach the script to the desired game object whose position you aim to control;
- Configuration: Within the script, specify the value or range where you’d like to clamp the Y-axis;
- Run & Observe: Play the game to witness the clamp in action.
using UnityEngine;
public class vector_clamp : MonoBehaviour
{
Vector3 pos;
float height_restriction = 10f;
void Start()
{
pos = transform.position;
}
void Update()
{
pos.y += 0.5f;
pos.y = Mathf.Clamp(pos.y, 0, height_restriction);
transform.position = pos;
}
}
For clamping the X or Z axes, the process is similar. Just adjust the axis of interest in the script.
3. Clamping Integer Values in Unity
The versatility of Unity’s Mathf.Clamp function extends to both floats and integers. This method returns a value limited between specified minimum and maximum boundaries. The data type of the output is determined by the data types of the specified range.
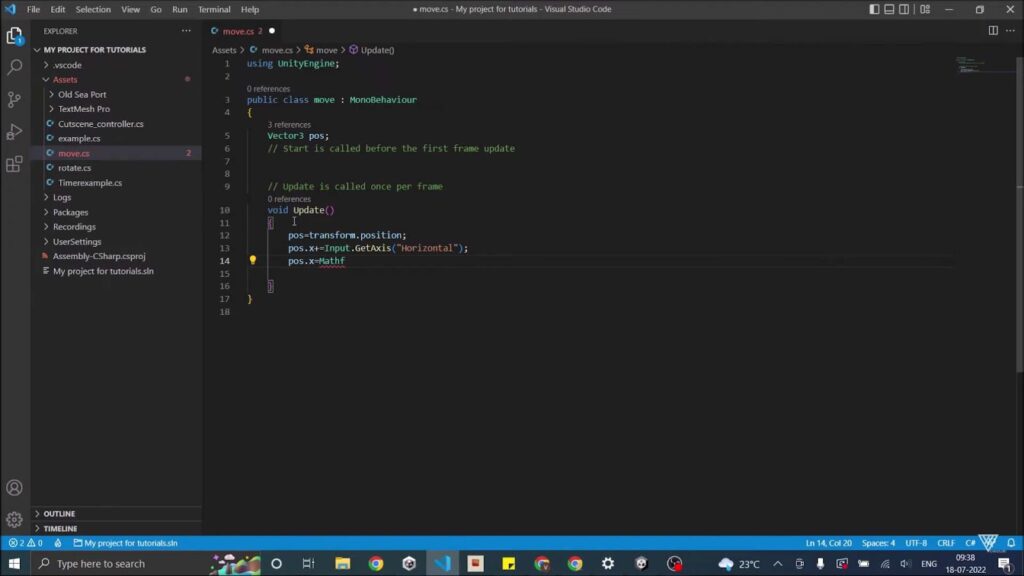
C# Example to Clamp an Integer
Here’s an illustrative script to demonstrate the clamping of an integer value:
using UnityEngine;
public class Mathf_example1 : MonoBehaviour
{
int my_value = 0;
int min_value = 10;
int max_value = 20;
void Update()
{
my_value += 1;
my_value = Mathf.Clamp(my_value, min_value, max_value);
Debug.Log(my_value);
}
}
In the above script, the key difference from a float-based clamp is the data type. Every parameter within the Mathf.Clamp method, in this instance, is of integer type, ensuring the clamped value remains an integer.
Conclusion
In conclusion, the Mathf.Clamp function in Unity C# is an essential tool in a game developer’s toolkit. It provides a simple and efficient way to ensure that values stay within a specified range, preventing unexpected behaviors and errors in your game code. Whether you’re controlling character movement, managing health points, or handling any other numerical parameter, Mathf.Clamp can help maintain control and predictability in your game mechanics.
In this tutorial, we’ve explored the basic syntax and usage of Mathf.Clamp, learning how to effectively constrain values within desired boundaries. By using this function, you can create more robust and user-friendly game systems, enhancing the overall player experience. Remember that mastering Mathf.Clamp is just one step toward becoming a proficient Unity programmer, and it can be combined with other Unity features to create even more complex and engaging gameplay.