If you’re a game developer using Unity, you’re probably familiar with the concept of prefabs and the need to instantiate them within your game world. This guide will take you through the essentials of instantiating prefabs in Unity, with and without code, providing you with a versatile toolset to enhance your game development skills.
Unity Instantiate Function
Before diving into the details of instantiating prefabs, it’s essential to understand the Unity Instantiate function. This function plays a pivotal role in spawning prefabs or game objects at any point in your game world. It takes four arguments, with only one being mandatory:
- Object: This argument represents the prefab or game object you want to spawn, and it’s the only mandatory input;
- Position: You can specify the Vector3 position where the object should spawn. If no position is provided, it will spawn at the location of the game object to which the Instantiate function is added;
- Rotation: This argument defines the Quaternion rotation of the spawned object. You can use Quaternion. Identity to spawn the object with zero rotation;
- Parent: If provided, this argument determines the transform of the object that will be set as the parent of the spawned game object.
Understanding Unity Prefab
Before you can instantiate a prefab, it’s crucial to grasp what a Unity prefab is. In Unity, a prefab is a game object that has been customized and prepared for deployment within a game scene. It allows you to set the position, rotation, scale, and other components, creating a template that can be easily spawned in your game at a later stage. Essentially, any game object in which you add the required components and keep it ready in the Unity resources folder for deployment is referred to as a prefab.
Instantiating a Prefab in Unity
Now, let’s walk through the steps to instantiate a prefab in Unity:
Step 1: Create a Prefab in Unity
- Open a new Unity project and navigate to the Hierarchy window;
- Create a new game object and add the necessary components to it. You can use the default Unity cube for this tutorial;
- To turn this game object into a prefab, drag and drop it from the Hierarchy window to the Project window. This action creates your prefab, and you can now safely delete the game object from the Hierarchy window.
Step 2: Adding Instantiate Script to the Scene
- Create an empty game object in the Hierarchy window;
- Add a new script component to this empty game object and name it “Instantiate_example.”;
- Open the script in Visual Studio or your preferred code editor and paste the provided code snippet.
using UnityEngine;
using System.Collections;
public class Instantiate_example: MonoBehaviour
{
public Transform prefab;
void Start()
{
Instantiate(prefab, new Vector3(2.0F, 0, 0), Quaternion.identity);
}
}
Step 3: Assign Prefab or Game Object to the Script
- Select the script object in the Hierarchy view;
- Drag and drop the prefab you created earlier onto the “prefab” variable in the Inspector window.
Now, when you run your game, the prefab will be instantiated into your game scene at the start. Unity, by default, names the instantiated game object as a clone of your prefab, appending “(Clone)” to the original name. For example, if your prefab is named “Friend Cube,” Unity will name it “Friend Cube(Clone).”
If you want to instantiate the object conditionally, such as on pressing the space key or clicking the mouse, you can move the instantiation logic to the Update method and use an if statement to trigger it.
Instantiate Prefab as a Child of Another Object
The Unity Instantiate function allows you to specify a parent object as the fourth argument. When you do this, the spawned game object becomes a child of the parent game object. Here’s an example script to spawn a game object as a child of another:
using UnityEngine;
public class Instantiate_example : MonoBehaviour
{
public Transform prefab;
public Transform parent;
void Start()
{
Instantiate(prefab, new Vector3(2.0F, 0, 0), Quaternion.identity, parent);
}
}
Instantiating a Prefab in Unity by Name
In some cases, you may want to instantiate a prefab by its name. To achieve this, you can reference the prefab game object and load it from the Resources folder using Resources.Load(). However, this method is less performant and not recommended for frequent use. Here’s a code snippet demonstrating how to do it:
using UnityEngine;
using System.Collections;
public class Instantiate_example : MonoBehaviour
{
void Start()
{
GameObject newPlayer = Instantiate(Resources.Load("Player", typeof(GameObject))) as GameObject;
}
}
Giving a Custom Name to the Instantiated Prefab
By default, when you instantiate an object in Unity, it receives a name in the format “Prefab_name(Clone).” If you wish to provide a custom name for the instantiated object, you can assign it to a game object. Here’s an example of setting the name of the instantiated object to “MyObject”:
GameObject mySpawned = Instantiate(prefab, transform.position, Quaternion.identity) as GameObject;
mySpawned.name = "MyObject";
Instantiating a Projectile in Unity
To instantiate a projectile in Unity, you need to add force to it so that it moves. Typically, you would create an empty game object and position it where you want the projectile to spawn. Here are the steps to achieve this:
- Create an empty game object and name it “Projectile_Spawner.”;
- Add a script component to it, and name the script “SpawnProjectile.”;
- Create a projectile prefab with a Rigidbody component, which is necessary to apply force to the projectile;
- Add the following script to instantiate the projectile and apply force to it:
using UnityEngine;
public class SpawnProjectile : MonoBehaviour
{
public GameObject projectile;
GameObject myProjectile;
Rigidbody rb;
float forceMagnitude = 5;
void Start()
{
myProjectile = Instantiate(projectile, transform.position, Quaternion.identity) as GameObject;
rb = myProjectile.GetComponent<Rigidbody>();
rb.AddForce(transform.forward * forceMagnitude, ForceMode.Impulse);
}
}
Assign your projectile prefab to the script, and you’ll be ready to launch projectiles within your game.
Using Components for Unity Instantiate
In the example of instantiating a projectile, we instantiated the whole prefab and then accessed the Rigidbody component by assigning it to another game object. However, there’s a more efficient way to do this. Instead of instantiating the entire game object, you can instantiate specific components directly, eliminating the need for the GetComponent function. This approach is more performance-efficient:
using UnityEngine;
public class SpawnProjectile : MonoBehaviour
{
public Rigidbody projectile;
Rigidbody spawnedProjectile;
float forceMagnitude = 5;
void Start()
{
spawnedProjectile = Instantiate(projectile, transform.position, Quaternion.identity);
spawnedProjectile.AddForce(transform.forward * forceMagnitude, ForceMode.Impulse);
}
}
How to Instantiate at Random Positions in Unity
To instantiate game objects at random positions in Unity, you can generate random numbers and create a new Vector3 with them. Typically, you’d keep the Y value constant and randomize the X and Z values. Here’s how you can achieve this using the Random.Range function:
using UnityEngine;
public class Instantiate_Random : MonoBehaviour
{
[SerializeField] GameObject unityInstantiateObject;
float xPos;
float zPos;
float minPos = 0;
float maxPos = 10;
void Update()
{
if (Input.GetMouseButtonDown(0))
{
xPos = Random.Range(minPos, maxPos);
zPos = Random.Range(minPos, maxPos);
Vector3 pos = new Vector3(xPos, 0, zPos);
Instantiate(unityInstantiateObject, pos, Quaternion.identity);
}
}
}
Instantiate Using Visual Scripting (Without Code) in Unity
If you’re not comfortable coding in Unity, you can achieve object instantiation using visual scripting. Follow these steps to spawn a game object without writing code:
Create a Flow Graph:
- Create a new empty object in the Hierarchy window and name it “instantiate_graph.”;
- Add a Script Machine component to it;
- Create a new graph and name it “instantiate_example.”
Create the Logic:
Now, you can set up the logic for object instantiation using visual nodes:
- Add a timer node, a get rotation node, and an instantiate game object node;
- Set the timer duration to 5 seconds;
- Connect the “Start” event to the timer’s “Start” input and the timer’s “Completed” event to the “Instantiate” node;
- Set the game object in the “Instantiate” node to your desired prefab;
- Use the “Get Rotation” node to get the rotation you want and connect it as an input to the “Rotation” parameter of the “Instantiate” node.
By following these steps, you can create a visual script that spawns an object after a specific time delay.
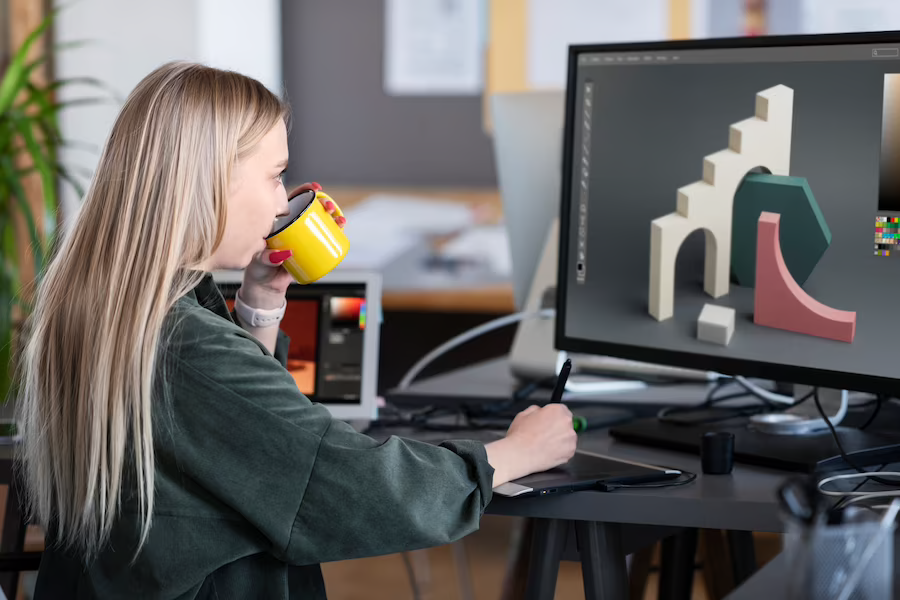
When and When Not to Use Unity Instantiate
While Unity’s Instantiate function is a powerful tool, it’s essential to use it judiciously to avoid memory issues and performance bottlenecks. Here are some guidelines on when to use Instantiate and when to consider alternative approaches:
Ideal Use Cases for Instantiate:
- Spawning Single Characters: It’s suitable for spawning individual characters that persist throughout the game, such as the player character;
- Limited Objects: Use to Instantiate for objects that are limited in number and are eventually destroyed, like ammo pickups, power-ups, etc;
- Temporary Effects: It’s appropriate for creating temporary effects on objects, like a fire that burns for a few seconds.
However, it’s crucial to remember that failing to destroy objects instantiated via Instantiate can lead to increased memory consumption and potential game freezes.
Alternative Approach: Object Pooling
For scenarios where you need to spawn multiple objects frequently, consider using object pooling instead of Instantiate. Object pooling involves creating a predefined pool of objects and activating and deactivating them as needed, instead of instantiating and destroying them. This approach reduces the memory overhead associated with Instantiate and improves game performance.
Comparative Table: Instantiate vs. Object Pooling in Unity
Aspect | Instantiate | Object Pooling |
---|---|---|
Performance | May cause performance issues when frequently used | Improved performance, especially for frequent spawns |
Memory Management | Consumes more memory due to instantiating and destroying objects | Efficient memory usage by reusing objects |
Initialization Overhead | High initialization overhead for each instantiation | Objects are pre-initialized and ready for use |
Object Reusability | Objects are not reused; new instances are created | Objects are recycled for reuse |
Implementation Complexity | Simpler to implement for occasional spawns | Requires more setup but better for frequent spawns |
Use Cases | Suitable for occasional spawns of unique objects | Ideal for frequent spawns, like bullets or enemies |
Example | Player character instantiation | Bullet or enemy instantiation |
Unity Functions | Use Instantiate function | Create and manage a custom object pool |
Video Explanation
In order to explain this topic in more detail we have prepared a special video for you. Enjoy watching it!
Conclusion
In conclusion, mastering the art of instantiating prefabs in Unity is a crucial skill for game developers. Whether you’re creating characters, projectiles, or dynamic effects, understanding how to spawn objects efficiently can significantly impact your game’s performance and overall player experience. By following the guidelines and examples provided in this guide, you’ll be well-equipped to handle object instantiation in Unity effectively.